In this example, I'll explain how I accessed my custom fields to used them to make a table using Google Charts API
OK, so the first thing you are going to need to do is create your own Model to expose the data required.
In my example, I created a Garage folder inside library. You will then need a ControllerPublic folder
/library/Garage/ControllerPublic
The file I created was called Garage.php, and this contains the functions needed.
The class used is Garage_ControllerPublic_Garage
I then have 3 functions used to get the various information I'm using (3 as I use this file for 3 different pages)
So taking the function getPowerBoard this is what will be used to pull the power figures from my custom field bhp
The below is the query and response we are going to pass back to the template
The two important lines of that are
WHERE xf_nflj_showcase_custom_field_value.field_id = 'bhp'
AND xf_nflj_showcase_custom_field_value.field_value != ''
which is the name of the custom field, and the AND statement makes it only pull results where there is data (ie not empty)
For the XenForo page, you want to create a page using a PHP callback to the Class and Function
This will then allow you access to the response params
The HTML used to make the page, which works with the Google Charts API
You want to loop through the results, with the below code:
<xen:foreach loop="$power" value="$power">
['<xen:username user="$power" />', '<a href="{xen:link showcase, $power}">{xen:jsescape {xen:raw $power.item_name}, single}</a>', '{$power.field_value} {$power.field_id}'],
</xen:foreach>
This links the user back to their profile, their vehicle to their showcase item, and pull the power value from the custom fields. You can see the $power.xxxx_xxxx which relates to the response and the specific field, ie $power.item_name
Hope this helps, any questions, please ask.
OK, so the first thing you are going to need to do is create your own Model to expose the data required.
In my example, I created a Garage folder inside library. You will then need a ControllerPublic folder
/library/Garage/ControllerPublic
The file I created was called Garage.php, and this contains the functions needed.
The class used is Garage_ControllerPublic_Garage
I then have 3 functions used to get the various information I'm using (3 as I use this file for 3 different pages)
PHP:
<?php
class Garage_ControllerPublic_Garage
{
public static function getPowerBoard(XenForo_ControllerPublic_Abstract $controller, XenForo_ControllerResponse_Abstract &$response)
{
$db = XenForo_Application::getDb();
$power = $db->fetchAll("
SELECT
xf_nflj_showcase_custom_field_value.*,
xf_nflj_showcase_item.item_id,
xf_nflj_showcase_item.user_id,
xf_nflj_showcase_item.item_name,
xf_user.user_id,
xf_user.username
FROM xf_nflj_showcase_custom_field_value
LEFT JOIN xf_nflj_showcase_item ON
(xf_nflj_showcase_custom_field_value.item_id = xf_nflj_showcase_item.item_id)
LEFT JOIN xf_user ON
(xf_nflj_showcase_item.user_id = xf_user.user_id)
WHERE xf_nflj_showcase_custom_field_value.field_id = 'bhp'
AND xf_nflj_showcase_custom_field_value.field_value != ''
ORDER BY xf_nflj_showcase_custom_field_value.field_value DESC
");
$response->params['power'] = $power;
}
public static function getCategory(XenForo_ControllerPublic_Abstract $controller, XenForo_ControllerResponse_Abstract &$response)
{
$db = XenForo_Application::getDb();
$category = $db->fetchAll("
SELECT
category_name,
item_count
FROM xf_nflj_showcase_category
");
$response->params['category'] = $category;
}
public static function getQuarterMile(XenForo_ControllerPublic_Abstract $controller, XenForo_ControllerResponse_Abstract &$response)
{
$db = XenForo_Application::getDb();
$power = $db->fetchAll("
SELECT
xf_nflj_showcase_custom_field_value.*,
xf_nflj_showcase_item.item_id,
xf_nflj_showcase_item.user_id,
xf_nflj_showcase_item.item_name,
xf_user.user_id,
xf_user.username
FROM xf_nflj_showcase_custom_field_value
LEFT JOIN xf_nflj_showcase_item ON
(xf_nflj_showcase_custom_field_value.item_id = xf_nflj_showcase_item.item_id)
LEFT JOIN xf_user ON
(xf_nflj_showcase_item.user_id = xf_user.user_id)
WHERE xf_nflj_showcase_custom_field_value.field_id = '14_Mile'
AND xf_nflj_showcase_custom_field_value.field_value != ''
ORDER BY xf_nflj_showcase_custom_field_value.field_value DESC
");
$response->params['power'] = $power;
}
}
So taking the function getPowerBoard this is what will be used to pull the power figures from my custom field bhp
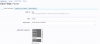
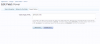
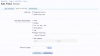
The below is the query and response we are going to pass back to the template
PHP:
$power = $db->fetchAll("
SELECT
xf_nflj_showcase_custom_field_value.*,
xf_nflj_showcase_item.item_id,
xf_nflj_showcase_item.user_id,
xf_nflj_showcase_item.item_name,
xf_user.user_id,
xf_user.username
FROM xf_nflj_showcase_custom_field_value
LEFT JOIN xf_nflj_showcase_item ON
(xf_nflj_showcase_custom_field_value.item_id = xf_nflj_showcase_item.item_id)
LEFT JOIN xf_user ON
(xf_nflj_showcase_item.user_id = xf_user.user_id)
WHERE xf_nflj_showcase_custom_field_value.field_id = 'bhp'
AND xf_nflj_showcase_custom_field_value.field_value != ''
ORDER BY xf_nflj_showcase_custom_field_value.field_value DESC
");
$response->params['power'] = $power;
The two important lines of that are
WHERE xf_nflj_showcase_custom_field_value.field_id = 'bhp'
AND xf_nflj_showcase_custom_field_value.field_value != ''
which is the name of the custom field, and the AND statement makes it only pull results where there is data (ie not empty)
For the XenForo page, you want to create a page using a PHP callback to the Class and Function
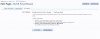
This will then allow you access to the response params
The HTML used to make the page, which works with the Google Charts API
HTML:
<style type="text/css">
.outercontainer {
border: 1px solid #BAC2C7;
padding: 10px;
margin: 0 auto;
background-color: #F4F6F7 !important;
}
</style>
<script type='text/javascript' src='https://www.google.com/jsapi'></script>
<script type='text/javascript'>
google.load('visualization', '1', {packages:['table']});
google.setOnLoadCallback(drawTable);
function drawTable() {
var data = new google.visualization.DataTable();
data.addColumn('string', 'Member');
data.addColumn('string', 'Vehicle');
data.addColumn('string', 'Power');
data.addRows([
<xen:foreach loop="$power" value="$power">
['<xen:username user="$power" />', '<a href="{xen:link showcase, $power}">{xen:jsescape {xen:raw $power.item_name}, single}</a>', '{$power.field_value} {$power.field_id}'],
</xen:foreach>
]);
var table = new google.visualization.Table(document.getElementById('table_div'));
table.draw(data, {showRowNumber: true, allowHtml: true});
}
</script>
<div class="outercontainer">
<div class="baseHtml">
<div id='table_div'></div>
</div>
</div>
You want to loop through the results, with the below code:
<xen:foreach loop="$power" value="$power">
['<xen:username user="$power" />', '<a href="{xen:link showcase, $power}">{xen:jsescape {xen:raw $power.item_name}, single}</a>', '{$power.field_value} {$power.field_id}'],
</xen:foreach>
This links the user back to their profile, their vehicle to their showcase item, and pull the power value from the custom fields. You can see the $power.xxxx_xxxx which relates to the response and the specific field, ie $power.item_name
Hope this helps, any questions, please ask.