The only other important thing relating to this which I think is needed is a better data validator which prompts to say which field has been entered incorrectly.
There is, however, it evaluates 1 at a time (in a loop) and when it finds the first one, it throws the error (so there may be more, but it won't know until the item is submitted again.
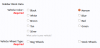
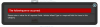